[JAVA] ResourceBundle로 다국어 메시지 처리
ResourceBundle이란?
프로그램을 만들 때, 다국어 처리(이하 i18n
)를 지원해야하는 경우가 있다. i18n
은 동일한 내용을 가진 메시지를 지역에 따라 서로 다른 언어로 메시지를 처리하는 것을 말한다. 예를 들어, 한국에서는 안녕하세요!
라고 보여주는 메시지를 영어를 쓰는 국가에서는 Hello!
로 보여줄 수 있도록 하는 것이 바로 i18n
다. Java
에서는 ResourceBundle
이라는 클래스를 사용해 i18n
을 지원한다.
ResourceBundle 사용하기
properties 파일 생성
i18n
지원을 위한 properties
파일 생성에는 규칙이 있는데, 그 규칙은 다음과 같다.
{프로퍼티명}_{언어코드}_{국가코드}.properties
{프로퍼티명}_{언어코드}.properties
{프로퍼티명}.properties
/*
* EX) 프로퍼티 명이 message이고, 미국일 경우
* message_en_US.properties
* message_en.properties
* message.properties
*/
ResourceBundle
는 실행될 때, 맨 위부터 아래의 작명 규칙대로 검색한다. 예를 들어 한국어로 작성된 properties
를 찾는다면, 가장 먼저 message_ko_KR.properties
를 검색할 것이고, 그 다음은 순차적으로 message_ko.properties
, message.properties
를 검색한다. 지원되지 않는 언어인 경우에는 ResourceBundle
이 최종적으로 message.properties
파일을 검색한다는 특성을 이용해, message.properties
에는 보통 미국 영어인 en_US
나 영국 영어 en_UK
가 많이 사용된다. 해당 파일은 main
의 resources
디렉토리 내부에 위치해야 한다.
ResourceBundle 로드
ResourceBundle resourceBundle = ResourceBundle.getBundle("message");
// ResourceBundle은 두 번째 인자로 지역을 지정할 수 있다.
ResourceBundle resourceBundle = ResourceBundle.getBundle("message", Locale.getDefault());
getBundle("message")
의 message
문자열은 프로퍼티 명이다. 만약 프로퍼티 명을 text
로 지정한다면, text_{언어코드}_{국가}.properties
를 검색하게 된다. 또한, 두 번째 인자로는 지역을 지정할 수 있는데, 두 번째 인자가 입력되면 해당 인자에 맞는 언어/국가코드를 ResourceBundle
이 자동으로 검색하게 된다. 참고로 위의 Locale.getDefault()
는 현재 실행중인 JVM
의 지역을 가리킨다.
만약 properties
파일들의 위치를 단계화 하고 싶다면, getBundle()
메소드의 인자를 단계화 하면 된다. 예를 들어 resource/messages/message.properties
파일을 검색하게 하고 싶다면 다음과 같이 작성하면 된다.
ResourceBundle resourceBundle = ResourceBundle.getBundle("messages/message");
프로퍼티 값 가져오기
message.properties
파일에 다음과 같은 내용이 있다고 가정하자.
key="value"
ResourceBundle
을 통해 value
값을 가져오고 싶다면, 다음과 같이 작성하면 된다.
ResourceBundle resourceBundle = ResourceBundle.getBundle("message");
String value = resourceBundle.getString("key");
System.out.println("key: " + value);
// 결과 -> key: value
정리하며
개인적으로 라이브러리를 개발하며, 에러 메시지 처리를 위해 i18n
를 공부 했었는데 이번에 기회가 되어 Java
에서 i18n
처리하는 방법을 정리해 보았다.
참고한 문헌 및 글
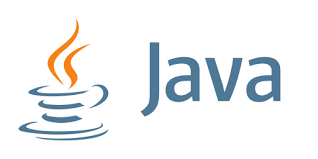